How to Read .dat Files in Matlab
And then far, we accept indirectly discussed several methods of getting input information from the user, and several methods of outputting the outcome in a MATLAB program. This note attempts at formalizing all the previous discussions and introduce more full general efficient methods of code interaction with users.
Methods of data input/output in MATLAB
Let'southward begin with an example code that explains the meaning of input/output (I/O) in MATLAB,
a = 0.1 ; b = 1 ; ten = 0.6 ; y = a * exp ( b * ten )
In the above code, a
, b
, x
are examples of input data to code, and y
is an instance of code output. In such cases as in the above, the input information is said to be hardcoded in the program.
Input data can be fed to a MATLAB program in four dissimilar ways,
- permit the user respond questions in a dialog in MATLAB terminal window,
- allow the user provide input on the operating organisation command line,
- let the user write input data in a graphical interface,
- let the user provide input data in a file.
At that place are ii major methods of data output,
- writing to the terminal window, as previously washed using
disp()
function, or, - writing to an output file.
We have already extensively discussed printing output to the terminal window. Reading from and writing data to file is also easy as we volition see here.
Input/output from MATLAB terminal window
We have already introduced and used this method frequently in the past, via the MATLAB's congenital-in function input()
. If we were to get the input data for the above code via the terminal window, an example approach would be the following,
datain = input ( 'input [a,b,c]: ' ); a = datain ( 1 ); b = datain ( 2 ); x = datain ( 3 ); y = a * exp ( b * x )
input a,b,c: [0.1, ane, 0.half-dozen] y = 0.1822
One could too read the input values as cord ans then catechumen them to real values or parse the input using one of MATLAB'southward congenital-in functions, for example,
>> datain = input ( 'input [a,b,c]: ' , 's' ); input [ a , b , c ]: [ 0.ane , 1 , 0.half dozen ] >> class ( datain )
>> datain = str2num ( datain )
datain = 0.one thousand 1.0000 0.6000
>> a = datain ( 1 ); >> b = datain ( 2 ); >> ten = datain ( iii ); >> y = a * exp ( b * 10 )
Input/output data from operating system's command line
This approach is almost popular in Unix-like environments, where well-nigh users are accustomed to using the Bash command line. However, it can be readily used in Windows cmd environment as well. For this approach, we accept to invoke MATLAB from the computer operating arrangement'due south control line, that is, Bash in Linux systems, and cmd in Windows,
kickoff matlab -nosplash -nodesktop -r "testIO"
Then a MATLAB control-line window opens in your calculator like the following that runs automatically your code (stored in testIO.k
).
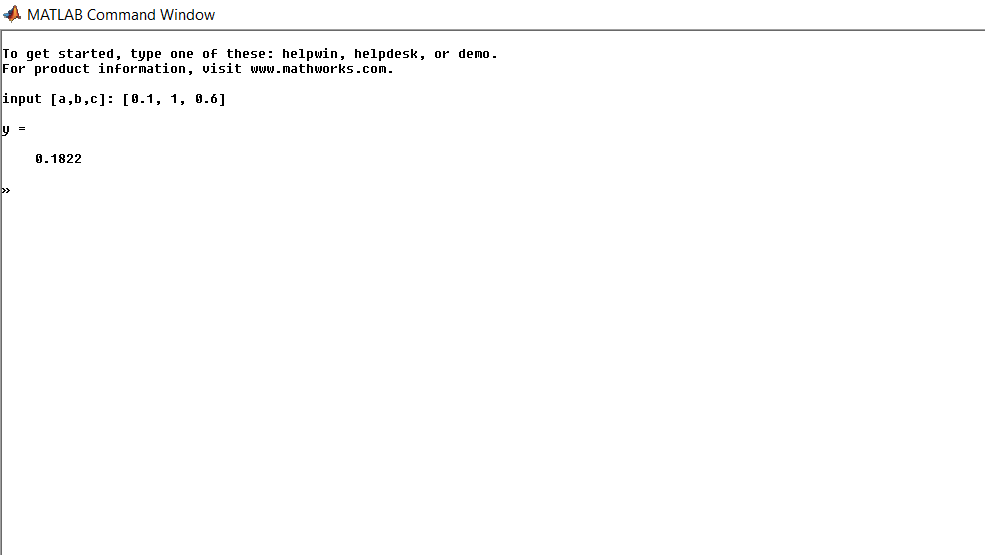
In the above control, we are starting MATLAB from the Os control line with our own choice of optional arguments for MATLAB. You can specify startup options (also called command flags or control-line switches) that instruct the MATLAB plan to perform sure operations when you offset it. On all platforms, specify the options as arguments to the MATLAB command when you lot start at the operating system prompt. For case, the following starts MATLAB and suppresses the display of the splash screen (a splash screen is a graphical control chemical element consisting of a window containing an prototype, a logo, and the current version of the software. A splash screen usually appears while a game or programme is launching),
The flag -nodesktop
issue in opening merely the MATLAB control line, and no MATLAB Graphical user interface (GUI) just like the figure above. Finally, the flag -r
executes the MATLAB file that appears right after it, specified as a string or equally the proper name of a MATLAB script or function. If a MATLAB statement is MATLAB lawmaking, you should enclose the string with double quotation marks. If a MATLAB statement is the proper noun of a MATLAB function or script, practise not specify the file extension and do not utilise quotation marks. Any required file must exist on the MATLAB search path or in the startup folder. Y'all can also set MATLAB'southward working folder right from the control-line using -sd
flag. You can observe observe more information about all possible flags here. On Windows platforms, you can precede a startup option with either a hyphen (-
) or a slash (/
). For instance, -nosplash
and /nosplash
are equivalent.
Note that y'all can also quote MATLAB on the Bone control line, forth with the name of the script y'all want to run. For case, suppose you wanted to run the original script,
a = 0.i ; b = 1 ; x = 0.six ; y = a * exp ( b * x )
However, now with a
, b
, x
, given at runtime. You could write a script file test.m
that contains,
and give the variables values at runtime, on Os command line, like the following,
matlab -nosplash -nodesktop -r "a = 0.1; b = 1; x = 0.6; testIO"
The figure below shows a screen-shot illustrating the output of the in a higher place command.
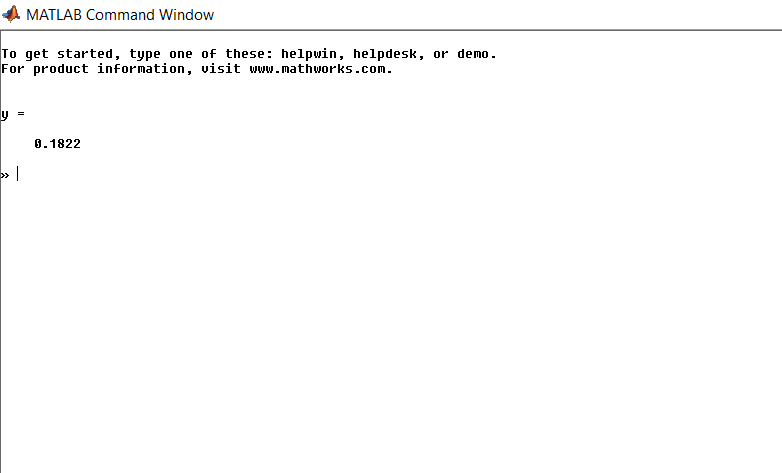
Input/output data from a Graphical User Interface
This method of inputting data is done by constructing a Graphical User Interface (GUI) which opens and takes input from the user. This is probably one of the almost convenient methods for users to input data. You can do this in MATLAB for example by using the built-in office inputdlg()
which creates a dialog box that gathers user input. But this method of data collection is beyond the scope of our class. More than information nigh this tin be found hither.
Input/output data from file
In cases where the input/output data is large, the command-line arguments and input from the terminal window are not efficient anymore. In such cases, the most common approach is to permit the lawmaking read/write data from a pre-existing file, the path to which is most often given to the code via the Os control line or MATLAB terminal window.
There are many methods of importing and exporting data to and from MATLAB, only some of which nosotros will discuss here. For more than information see here, here, and here. The following tabular array shows some of the near important import functions in MATLAB, which we volition discuss here every bit well.
Part | Description |
---|---|
load() | Load MATLAB variables from file into MATLAB workspace |
salve() | save MATLAB variables from MATLAB workspace into a MATLAB `.mat` file. |
fscanf() | Read data from text file |
fprintf() | Write information to a text file |
dlmread() | Read ASCII-delimited file of numeric data into matrix |
dlmwrite() | Write a numeric matrix into ASCII-delimited file |
csvread() | Read comma-separated value (CSV) file |
csvwrite() | Write values of a matrix into a comma-separated (CSV) file |
xlswrite() | Read Microsoft Excel spreadsheet file |
xlswrite() | write information into a Microsoft Excel spreadsheet file |
readtable() | Create table from file |
writetable() | Write table to file |
imread() | Read image from graphics file |
imwrite() | Write image to graphics file |
importdata() | Load data from file |
textscan() | Read formatted data from text file or string |
fgetl() | Read line from file, removing newline characters |
fread() | Read information from binary file |
fwrite() | Write data to binary file |
type() | Display contents of file |
Loading/saving MATLAB workspace variables
MATLAB has two useful functions that can save the workspace variables into special MATLAB .mat
files, to be afterward load again into the same or another MATLAB workspace for further piece of work or manipulation. The function save()
saves workspace variables to a given file. The most useful options for this function are the following,
save ( filename ) save ( filename , variables ) save ( filename , variables , fmt )
-
save(filename)
saves all variables from the current workspace in a MATLAB formatted binary file called MAT-file with the given proper namefilename
. If the filefilename
exists,save()
overwrites the file. -
save(filename,variables)
saves merely the variables or fields of a construction assortment specified byvariables
. For example,p = rand ( 1 , 10 ); q = ones ( 10 ); save ( 'pqfile.mat' , 'p' , 'q' )
volition create the binary MAT file pqfile.mat which contains the two variables.
-
salvage(filename,variables,fmt)
saves the requested variables with the file format specified byfmt
. The variables argument is optional. If you lot do not specify variables, the save function saves all variables in the workspace. File format, specified as one of the following. When using the command course of save, you do not need to enclose the input in single or double quotes, for case,save myFile.txt -ascii -tabs
. For instance,p = rand ( 1 , 10 ); q = ones ( 10 ); save ( 'pqfile.txt' , 'p' , 'q' , '-ascii' )
will create an ASCII text file pqfile.txt which contains the two variables
p
andq
.
Value of fmt | File Format |
---|---|
'-mat' | Binary MAT-file format. |
'-ascii' | Text format with 8 digits of precision. |
'-ascii','-tabs' | Tab-delimited text format with 8 digits of precision. |
'-ascii','-double' | Text format with 16 digits of precision. |
'-ascii','-double','-tabs' | Tab-delimited text format with 16 digits of precision. |
Similarly, one tin reload the same files into MATLAB workspace again if needed, for instance using MATLAB load()
function,
>> load ( 'pqfile.txt' ) >> pqfile
pqfile = Columns ane through 8 0.0975 0.2785 0.5469 0.9575 0.9649 0.1576 0.9706 0.9572 1.0000 one.0000 ane.0000 ane.0000 ane.0000 1.0000 i.0000 i.0000 i.0000 1.0000 1.0000 1.0000 1.0000 1.0000 1.0000 1.0000 one.0000 1.0000 1.0000 1.0000 ane.0000 1.0000 1.0000 one.0000 1.0000 i.0000 ane.0000 1.0000 1.0000 one.0000 1.0000 1.0000 ane.0000 i.0000 1.0000 1.0000 1.0000 ane.0000 1.0000 1.0000 ane.0000 1.0000 1.0000 1.0000 1.0000 1.0000 1.0000 1.0000 i.0000 ane.0000 1.0000 1.0000 1.0000 1.0000 1.0000 i.0000 ane.0000 ane.0000 1.0000 i.0000 i.0000 ane.0000 one.0000 ane.0000 1.0000 ane.0000 1.0000 1.0000 1.0000 1.0000 1.0000 1.0000 1.0000 1.0000 1.0000 1.0000 1.0000 ane.0000 ane.0000 ane.0000 Columns 9 through x 0.4854 0.8003 ane.0000 1.0000 1.0000 1.0000 one.0000 1.0000 i.0000 i.0000 1.0000 one.0000 one.0000 1.0000 one.0000 i.0000 one.0000 1.0000 ane.0000 1.0000 1.0000 i.0000
Simply annotation that upon loading the Ascii file, the data about the individual variables is lost. By contrast, loading data using the MAT file will preserve the structure of the variables,
>> load ( 'pqfile.mat' ) >> p
p = Columns ane through 8 0.1419 0.4218 0.9157 0.7922 0.9595 0.6557 0.0357 0.8491 Columns 9 through 10 0.9340 0.6787
q = 1 1 1 1 i 1 1 1 one i one 1 1 i 1 one 1 1 1 1 1 1 i ane 1 1 1 i 1 1 1 ane i i 1 1 1 ane i one ane i 1 1 1 1 1 1 ane 1 1 one 1 one 1 1 i 1 one ane 1 ane 1 one ane 1 1 one 1 1 1 1 1 1 1 1 1 one 1 1 ane one 1 1 1 1 1 ane 1 i 1 one one 1 1 one ane 1 1 1
Reading/writing a formatted file using fscanf()
and fprintf()
There are numerous methods of reading the contents of a file in MATLAB. The most piffling and probably least pleasing method is through MATLAB's built-in office fscanf()
. To read a file, say this file, you lot will take to first open information technology in MATLAB,
fileID = fopen ( 'data.in' , 'r' ); formatSpec = '%f' ; A = fscanf ( fileID , formatSpec ) fclose ( fileID );
Note that unlike the C language'due south fscanf()
, in MATLAB fscanf()
is vectorized meaning that information technology can read multiple lines all at once. Here, the aspect 'r'
states that the file is opened to read it (vs writing, or another purpose). A list of available options for fopen()
is the following,
Attribute | Clarification |
---|---|
'r' | Open file for reading. |
'w' | Open up or create new file for writing. Discard existing contents, if any. |
'a' | Open up or create new file for writing. Append data to the end of the file. |
'r+' | Open up file for reading and writing. |
'west+' | Open or create new file for reading and writing. Discard existing contents, if whatever. |
'a+' | Open or create new file for reading and writing. Append information to the end of the file. |
'A' | Open file for appending without automatic flushing of the current output buffer. |
'W' | Open file for writing without automatic flushing of the current output buffer. |
The general syntax for reading an assortment from an input file using fscanf()
is the following,
array = fscanf ( fid , format ) [ array , count ] = fscanf ( fid , format , size )
where the optional argument size
specifies the amount of information to be read from the file. There are three versions of this statement,
-
n
: Reads exactlynorthward
values. Later on this statement,array
volition be a column vector containingnorthward
values read from the file. -
Inf
: Reads until the terminate of the file. After this argument,assortment
will be a column vector containing all of the data until the end of the file. -
[n m]
: Reads exactly, $n\times m$ values, and format the information as an $due north\times k$ assortment. For example, consider this file, which contains two columns of numeric data. One could read this data usingfscanf()
like the post-obit,>> formatSpec = '%d %f' ; >> sizeA = [ two Inf ]; >> fileID = fopen ( 'nums2.txt' , 'r' ); >> A = transpose ( fscanf ( fileID , formatSpec , sizeA )) >> fclose ( fileID );
A = 1.0000 2.0000 3.0000 4.0000 v.0000 0.8147 0.9058 0.1270 0.9134 0.6324
Now suppose you perform some on operation on A
, say the elemental multiplication of A
by itself. Then you want to shop (append) the result into another file. Yous can do this using MATLAB function fprintf()
,
>> formatSpec = '%d %f \n' ; >> fileID = fopen ( 'nums3.txt' , 'w+' ); >> fprintf ( fileID , formatSpec , A .* A ); >> fclose ( fileID );
The option w+
tells MATLAB to store the result in a file named num3.txt, and if the file does already exist, and so append the issue to the end of the electric current existing file. To see what formatting specifiers y'all tin utilize with MATLAB fscanf()
and fprintf()
, see this page.
MATLAB as well has some rules to skip characters that are unwanted in the text file. These rules are really details that are specific to your needs and the best arroyo is to seek the solution to your specific trouble past searching MATLAB'due south manual or the web. For instance, consider this file which contains a set of temperature values in degrees (including the Celsius degrees symbol). One manner to read this file and skipping the degrees symbol in MATLAB could be so the following set up of commands,
>> fileID = fopen ( 'temperature.dat' , 'r' ); >> degrees = char ( 176 ); >> [ A , count ] = fscanf ( fileID , [ '%d' degrees 'C' ]) >> fclose ( fileID );
A = 78 72 64 66 49 count = 5
This method of reading a file is very powerful but rather detailed, low-level and cumbersome, especially that you have to define the format for the content of the file appropriately. Most frequently, other higher-level MATLAB's congenital-in role come to rescue u.s.a. from the hassles of using fscanf()
. For more data most this function though, if you really desire to stick to it, see here. Some important MATLAB special characters (escape characters) that can as well appear in fprintf()
are also given in the following table.
Symbol | Effect on Text |
---|---|
'' | Single quotation mark |
%% | Single per centum sign |
\\ | Unmarried backslash |
\due north | New line |
\t | Horizontal tab |
\5 | Vertical tab |
Reading/writing data using dlmread()/dlmwrite()
and csvread()/csvwrite()
The methods discussed to a higher place are rather primitive, in that they require a scrap of effort by the user to know something nigh the structure of the file and its format. MATLAB has a long list of advanced IO functions that tin can handle a wide variety of data file formats. 2 of the most common functions are dedicated specifically to read data files containing delimited data sets: csvread()
and dlmread()
.
In the field of scientific calculating, a Comma-Separated Values (CSV) data file is a type of file with extension .csv
, which stores tabular data (numbers and text) in plain text format. Each line of the file is called a data record and each record consists of one or more fields, separated by commas. The utilise of the comma equally a field separator is the source of the name for this file format.
Now suppose you wanted to read two matrices whose elements were stored in CSV format in 2 csv data files matrix1.csv and matrix2.csv. You can achieve this task only past calling MATLAB'due south born csv-reader role called csvread(filename)
. Here the word filename
is the path to the file in your local difficult bulldoze. For case, download these 2 given csv files above in your MATLAB working directory and so try,
>> Mat1 = csvread ( 'matrix1.csv' ); >> Mat2 = csvread ( 'matrix2.csv' );
Then suppose yous want to multiply these two vectors and store the result in a new variable and write it to a new output CSV file. You could do,
Mat3 = Mat1 * Mat2 ; >> csvwrite ( 'matrix3.csv' , Mat3 )
which would output this file: matrix3.csv for you.
Alternatively, you could also employ MATLAB's built-in functions dlmread()
and dlmwrite()
functions to do the same things as above. These two functions read and write ASCII-delimited file of numeric data. For example,
>> Mat1 = dlmread ( 'matrix1.csv' ); >> Mat2 = dlmread ( 'matrix2.csv' ); >> Mat3 = Mat1 * Mat2 ; >> dlmwrite ( 'matrix3.dat' , Mat3 );
Notation that, dlmread()
and dlmwrite()
come with an optional statement delimiter
of the following format,
>> dlmread ( filename , delimiter ) >> dlmwrite ( filename , matrixObject , delimiter )
where the statement delimiter
is the field delimiter character, specified as a character vector or string. For example, in the above case, the delimiter is comma ','
. In other cases, yous could, for example, use white space ' '
, or '\t'
to specify a tab delimiter, and then on. For instance, you lot could have equally written,
>> dlmwrite ( 'matrix4.dat' , Mat3 , '\t' );
to create a tab-delimited file named matrix4.dat.
Reading/writing data using xlsread()
and xlswrite()
Once data becomes more than complex than simple numeric matrices or vectors, then we need more circuitous MATLAB functions for IO. An example of such a case is when you take stored your information in a Microsoft Excel file. For such cases, you can use xlsread(filename)
to read the file specified by the input argument filename
to this role. We volition, subsequently on, see some example usages of this role in homework. Similarly, yous could write data into an excel file using xlswrite()
. For example,
>> values = { 1 , 2 , 3 ; four , 5 , 'x' ; 7 , eight , nine }; >> headers = { 'Starting time' , 'Second' , 'Third' }; >> xlswrite ( 'XlsExample.xlsx' ,[ headers ; values ]);
would create this Microsoft Excel file for yous.
Reading/writing data using readtable()
and writetable()
Some other important and highly useful set of MATLAB functions for IO are readtable()
and writetable()
. The function readtable()
is used to read data into MATLAB in the class of a MATLAB table data type. For instance, you could read the same Excel file that we created above into MATLAB using readtable()
instead of xlsread()
,
>> XlsTable = readtable ( 'XlsExample.xlsx' )
XlsTable = Kickoff Second Third _____ ______ _____ 1 2 '3' 4 5 'ten' vii 8 '9'
Reading and writing prototype files using imread()
and imwrite()
MATLAB has a really broad range of input/output methods of information. We have already discussed some of the nigh useful IO approaches in the previous sections. For graphics files, even so, none of the previous functions are useful. Suppose you wanted to import a jpg or png or some other type graphics file into MATLAB to further process it. For this purpose, MATLAB has the congenital-in office imread()
which tin can read image from an input graphics file. For example, to read this image file in MATLAB, you lot could do,
>> homer = imread ( 'homer.jpg' ); >> imshow ( homer )
to get the following figure in MATLAB,
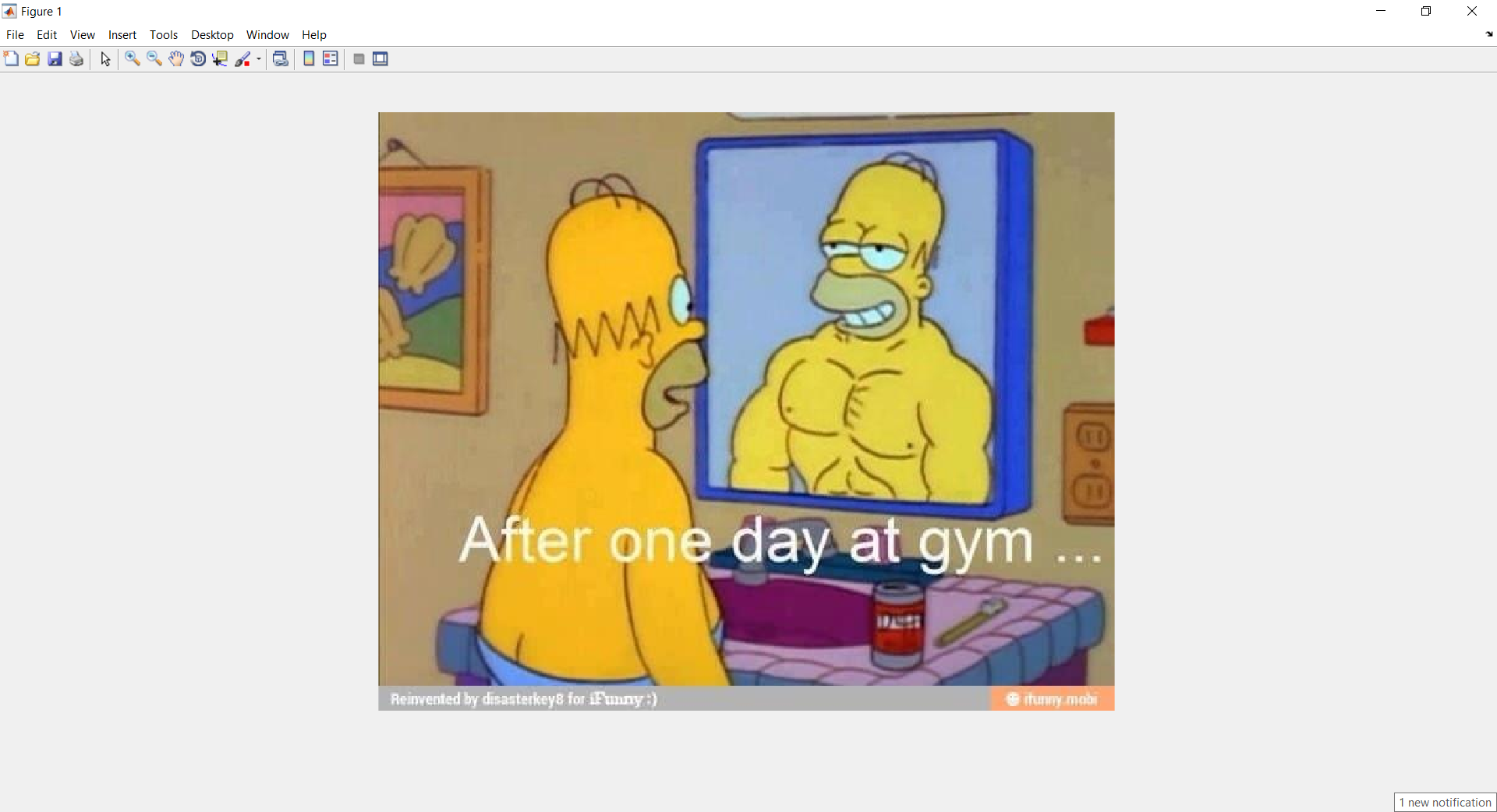
Now suppose you want to convert this figure to black-and-white and salvage information technology as a new figure. You could exercise,
>> homerBW = rgb2gray ( homer ); >> imshow ( homerBW ) >> imwrite ( homerBW , 'homerBW.png' );
to get this black and white version of the in a higher place image, at present in png format (or in whatever format you may wish, that is also supported by MATLAB).
Reading a file using importdata()
Probably, the well-nigh general MATLAB function for data input is importdata()
. This part can be used to import almost any blazon of data and MATLAB is capable of automatically recognizing the right format for reading the file, based on its extension and content. For instance, you could read the same image file above, using importdata()
,
>> newHomer = importdata ( 'homer.jpg' ); >> imshow ( newHomer )
to import it to MATLAB. At the same fourth dimension, you could also use it to import information from the excel file that nosotros created above, XlsExample.xlsx,
>> newXls = importdata ( 'XlsExample.xlsx' )
newXls = information: [3x3 double] textdata: {3x3 jail cell} colheaders: {'First' '2d' 'Third'}
or similarly, read a csv-delimited file similar matrix3.csv,
>> newMat3 = importdata ( 'matrix3.csv' )
newMat3 = Columns 1 through seven 62774 103230 77362 87168 65546 64837 100700 104090 143080 104700 116500 108250 105400 111110 80351 112850 89506 113890 106030 70235 110620 99522 134130 73169 134190 117710 92878 94532 59531 102750 91679 111350 80539 84693 96078 58504 76982 52076 91449 80797 69246 61569 76170 104310 93950 114860 89779 101530 87014 91610 118380 90636 107840 91120 90247 84871 85943 110670 73451 114410 100840 111660 77908 82570 94427 57213 81175 79305 78718 68662 Columns eight through 10 79446 78102 106570 102950 116850 137810 113210 108800 128700 93013 119130 132700 95750 100980 100450 67044 80635 78006 86355 103760 119710 92649 98589 132660 73117 109270 99401 65283 66888 114030
In general, yous can use importdata()
to read MATLAB binary files (MAT-files), ASCII files and Spreadsheets, as well as images and sound files.
Reading a file using fgetl()
Some other useful MATLAB role for reading the content of a file is fgetl()
which can read a file line by line, removing the new line characters \n
from the end of each line. The entire line is read every bit a string. For example, consider this file. One could read the content of this text file using the function fgetl()
like the following,
>> fid = fopen ( 'text.txt' ); >> line = fgetl ( fid ) % read line excluding newline character
line = The main benefit of using a weakly-typed language is the ability to exercise rapid prototyping. The number of lines of code required to declare and use a dynamically allocated array in C (and properly clean upward later its use) is much greater than the number of lines required for the same procedure in MATLAB.
>> line = fgetl ( fid ) % read line excluding newline grapheme
>> line = fgetl ( fid ) % read line excluding newline character
line = Weak typing is also good for code-reuse. You can code a scalar algorithm in MATLAB and with relatively trivial effort modify it to piece of work on arrays as well equally scalars. The fact that MATLAB is a scripted instead of a compiled language also contributes to rapid prototyping.
Reading data from web using webread()
In today's world, information technology oft happens that the data you need for your research is already stored somewhere on the globe-wide-web. For such cases, MATLAB has built-in methods and functions to read and import data or fifty-fifty a webpage. For example, consider this page on this class'southward website. It is indeed a text file containing a prepare of IDs for some astrophysical events. Suppose, y'all needed to read and store these IDs locally on your device. Y'all could simply try the following lawmaking in MATLAB to fetch all of the table'south data in a single string via,
>> webContent = webread ( 'https://www.cdslab.org/matlab/notes/information-transfer/io/triggers.txt' )
webContent = '00745966 00745090 00745022 00744791 00741528 00741220 00739517 00737438 ... 00100319'
Now if nosotros wanted to get the individual IDs, we could just apply strplit()
function to split the IDs at the line pause characters '\n'
,
>> webContent = strsplit ( webContent , '\northward' )
webContent = i×1019 cell array Columns 1 through 11 {'00745966'} {'00745090'} {'00745022'} ...
Source: https://www.cdslab.org/matlab/notes/data-transfer/io/index.html